Swift5でUIBezierPathで線、三角、四角、円、楕円、円弧の描き方【Swift/Objective-C】
UIBezierPathを使用したパス描画による様々な図形の描画方法をご紹介します。
もくじ
UIBezierPathを使用するための準備
UIBezierPathを使用するためにdraw()メソッドの用意されたViewを作成する必要があります。
今回はDrawViewという命名でUIViewを継承したViewクラスを作成しました。
Swift5
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let drawView = DrawView(frame: self.view.bounds)
self.view.addSubview(drawView)
}
}
import UIKit
class DrawView: UIView {
override init(frame: CGRect) {
super.init(frame: frame);
self.backgroundColor = UIColor.clear;
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func draw(_ rect: CGRect) {
// ここにUIBezierPathを記述する
}
}
Objective-C
#import "ViewController.h"
#import "DrawView.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view, typically from a nib.
DrawView *drawView = [[DrawView alloc] initWithFrame:self.view.bounds];
[self.view addSubview:drawView];
}
@end
#import "DrawView.h"
@implementation DrawView
- (id)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
self.backgroundColor = [UIColor clearColor];
}
return self;
}
- (void)drawRect:(CGRect)rect {
// ここにUIBezierPathを記述する
}
@end
線の描き方
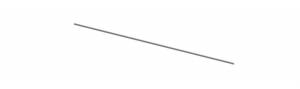
Swift5
// 線
let line = UIBezierPath()
// 最初の位置
line.move(to: CGPoint(x: 100, y: 100))
// 次の位置
line.addLine(to:CGPoint(x: 300, y: 150))
// 終わる
line.close()
// 線の色
UIColor.gray.setStroke()
// 線の太さ
line.lineWidth = 2.0
// 線を塗りつぶす
line.stroke()
点線の場合
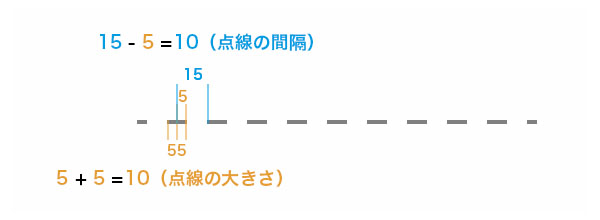
// 点線の大きさ, 点線の隙間
let dashes : [CGFloat] = [ 5, 15]
// 第一引数 点線の大きさ(数値*2になる), 点線の間隔(間隔-大きさになる)
// 第二引数 第一引数で指定した配列の要素数
// 第三引数 開始位置
line.setLineDash( dashes, count: dashes.count, phase: 0)

let dashes : [CGFloat] = [ 5, 15, 30]
点線をこのようにすることも可能
線、点線を角丸にする方法

line.lineCapStyle = .round
Objective-C
// 線
UIBezierPath *line = [UIBezierPath bezierPath];
// 最初の位置
[line moveToPoint:CGPointMake( 100, 100)];
// 次の位置
[line addLineToPoint:CGPointMake( 300, 150)];
// 終わる
[line closePath];
// 線の色
[[UIColor grayColor] set];
// 線の太さ
[line setLineWidth:2.0f];
// 線を塗りつぶす
[line stroke]
三角形の描き方
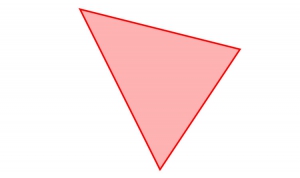
Swift5
// 三角形
let triangle = UIBezierPath()
triangle.move(to: CGPoint(x: 100, y: 100))
triangle.addLine(to:CGPoint(x: 300, y: 150))
triangle.addLine(to:CGPoint(x: 200, y: 300))
triangle.addLine(to:CGPoint(x: 100, y: 100))
triangle.close()
// 内側の色
UIColor(red: 1, green: 0, blue: 0, alpha: 0.3).setFill()
// 内側を塗りつぶす
triangle.fill()
// 線の色
UIColor(red: 1, green: 0, blue: 0, alpha: 1.0).setStroke()
// 線の太さ
triangle.lineWidth = 2.0
// 線を塗りつぶす
triangle.stroke()
Objective-C
// 三角形
UIBezierPath *triangle = [UIBezierPath bezierPath];
[triangle moveToPoint:CGPointMake( 100, 100)];
[triangle addLineToPoint:CGPointMake( 300, 150)];
[triangle addLineToPoint:CGPointMake( 200, 300)];
[triangle addLineToPoint:CGPointMake( 100, 100)];
[line closePath];
// 内側の色
[[UIColor colorWithRed:1 green:0 blue:0 alpha:0.3] setFill];
// 内側を塗りつぶす
[triangle fill];
// 線の色
[[UIColor colorWithRed:1 green:0 blue:0 alpha:1] set];
// 線の太さ
[triangle setLineWidth:2.0f];
// 線を塗りつぶす
[triangle stroke];
四角形の描き方
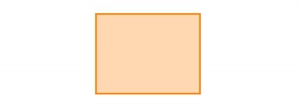
Swift5
let rectangle = UIBezierPath(rect: CGRect(x: 100, y: 100, width: 130, height: 100))
// 内側の色
UIColor(red: 1, green: 0.5, blue: 0, alpha: 0.3).setFill()
// 内側を塗りつぶす
rectangle.fill()
// 線の色
UIColor(red: 1, green: 0.5, blue: 0, alpha: 1.0).setStroke()
// 線の太さ
rectangle.lineWidth = 2.0
// 線を塗りつぶす
rectangle.stroke()
Objective-C
// 四角形(短形)
UIBezierPath *rectangle = [UIBezierPath bezierPathWithRect:CGRectMake(100, 100, 130, 100)];
// 内側の色
[[UIColor colorWithRed:1 green:0.5 blue:0 alpha:0.3] setFill];
// 内側を塗りつぶす
[rectangle fill];
// 線の色
[[UIColor colorWithRed:1 green:0.5 blue:0 alpha:1.0] setStroke];
// 線の太さ
[rectangle setLineWidth:2.0f];
// 線を塗りつぶす
[rectangle stroke];
角が丸い四角形の描き方
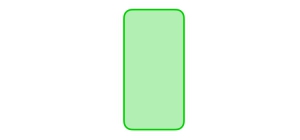
Swift5
// 角が丸い四角形(短形)
let roundrRectangle = UIBezierPath(roundedRect: CGRect(x: 50, y: 370, width: 75, height: 150), cornerRadius: 10.0)
// 内側の色
UIColor(red: 0, green: 0.8, blue: 0, alpha: 0.3).setFill()
// 内側を塗りつぶす
roundrRectangle.fill()
// 線の色
UIColor(red: 0, green: 0.8, blue: 0, alpha: 1.0).setStroke()
// 線の太さ
roundrRectangle.lineWidth = 2.0
// 線を塗りつぶす
roundrRectangle.stroke()
Objective-C
// 角が丸い四角形
UIBezierPath *roundrRectangle = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(50, 370, 75, 150) cornerRadius:10.0f];
// 内側の色
[[UIColor colorWithRed:0 green:0.8 blue:0 alpha:0.3] setFill];
// 内側を塗りつぶす
[roundrRectangle fill];
// 線の色
[[UIColor colorWithRed:0 green:0.8 blue:0 alpha:1.0] setStroke];
// 線の太さ
[roundrRectangle setLineWidth:2.0f];
// 線を塗りつぶす
[roundrRectangle stroke];
円の描き方
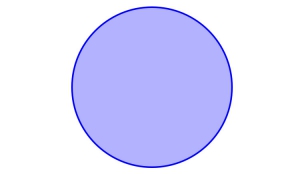
Swift5
// 円
let circle = UIBezierPath(arcCenter: CGPoint(x: 150, y: 150), radius: 100, startAngle: 0, endAngle: CGFloat(Double.pi)*2, clockwise: true)
// 内側の色
UIColor(red: 0, green: 0, blue: 1, alpha: 0.3).setFill()
// 内側を塗りつぶす
circle.fill()
// 線の色
UIColor(red: 0, green: 0, blue: 1, alpha: 1.0).setStroke()
// 線の太さ
circle.lineWidth = 2.0
// 線を塗りつぶす
circle.stroke()
Objective-C
// 円
UIBezierPath *circle = [UIBezierPath bezierPathWithArcCenter:CGPointMake(250, 250) radius:100 startAngle:0 endAngle:M_PI*2 clockwise:YES];
// 内側の色
[[UIColor colorWithRed:0 green:0 blue:1 alpha:0.3] setFill];
// 内側を塗りつぶす
[circle fill];
// 線の色
[[UIColor colorWithRed:0 green:0 blue:1 alpha:1.0] setStroke];
// 線の太さ
[circle setLineWidth:2.0f];
// 線を塗りつぶす
[circle stroke];
楕円の描き方
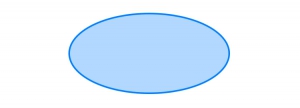
Swift5
// 楕円
let oval = UIBezierPath(ovalIn: CGRect(x: 100, y: 200, width: 200, height: 100))
// 内側の色
UIColor(red: 0, green: 0.5, blue: 1, alpha: 0.3).setFill()
// 内側を塗りつぶす
oval.fill()
// 線の色
UIColor(red: 0, green: 0.5, blue: 1, alpha: 1.0).setStroke()
// 線の太さ
oval.lineWidth = 2.0
// 線を塗りつぶす
oval.stroke()
Objective-C
// 楕円
UIBezierPath *oval = [UIBezierPath bezierPathWithOvalInRect:CGRectMake( 100, 200, 200, 100)];
// 内側の色
[[UIColor colorWithRed:0 green:0.5 blue:1 alpha:0.3] setFill];
// 内側を塗りつぶす
[oval fill];
// 線の色
[[UIColor colorWithRed:0 green:0.5 blue:1 alpha:1.0] setStroke];
// 線の太さ
[oval setLineWidth:2.0f];
// 線を塗りつぶす
[oval stroke];
円弧の描き方
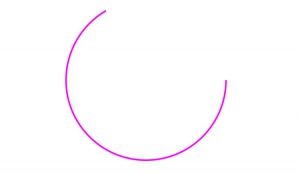
Swift5
// 円弧
let circle = UIBezierPath(arcCenter: CGPoint(x: 200, y: 200), radius: 100, startAngle: 0, endAngle: CGFloat(Double.pi)*4/3, clockwise: true)
// 線の色
UIColor(red: 1, green: 0, blue: 1, alpha: 1.0).setStroke()
// 線の太さ
circle.lineWidth = 2.0
// 線を塗りつぶす
circle.stroke()
Objective-C
// 円弧
UIBezierPath *arc = [UIBezierPath bezierPathWithArcCenter:CGPointMake(200, 200) radius:100 startAngle:0 endAngle:M_PI*4/3 clockwise:YES];
// 線の色
[[UIColor colorWithRed:1 green:0 blue:1 alpha:1.0] setStroke];
// 線の太さ
[arc setLineWidth:2.0f];
// 線を塗りつぶす
[arc stroke];
応用
UIBezierPathを使用した応用プログラムをご紹介します。