【Swift5】UITabBarの画像・文字の色・フォントを個別に設定する方法【Objective-C】
UITabBarの画像・文字の色・フォントを個別に設定する方法をご紹介します。
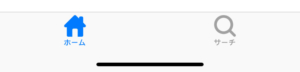
UITabBarの画像・文字の色はデフォルトでは単色です。
また、選択時は青、未選択時はグレーがデフォルトカラーとなっています。
今回ご紹介するUITabBarの画像・文字の色・フォントを個別に設定する方法はUITabBarを使うデザインの上でベストプラクティスと言えるぐらいカスタマイズ性が高い実装方法になります。
UITabBarを使用したアプリを作る場合はぜひこの実装をお試しください。
UITabBarの画像・文字の色・フォントを個別に設定する方法
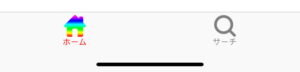
画像、文字を個別に設定するためにまず画像をon・offの状態で用意します。




tab_home_off@3x.png
tab_home_on@3x.png
tab_search_off@3x.png
tab_search_on@3x.png
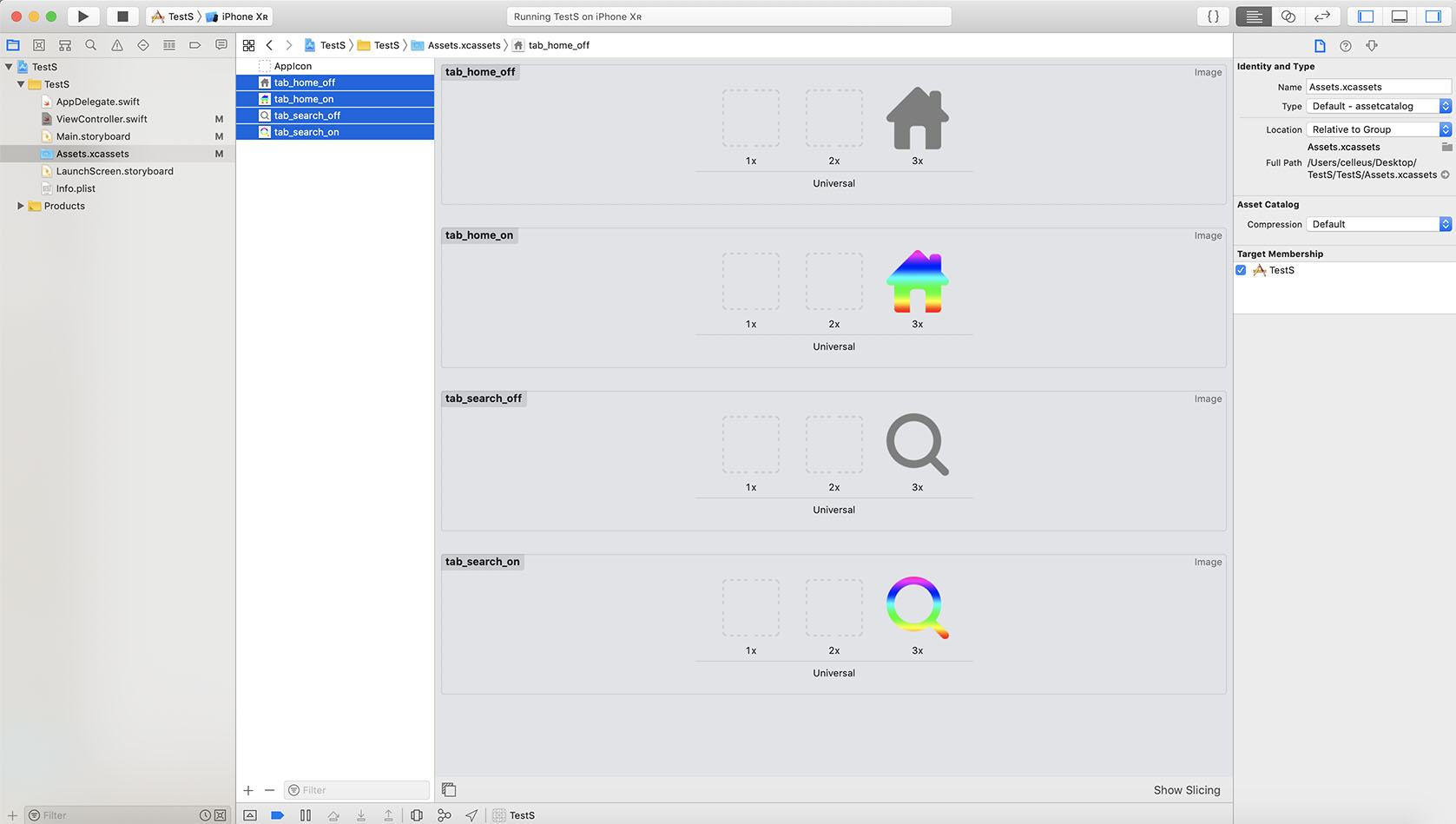
画像が用意できたらAssets.xcassetsに追加します。
これで下準備は完了です。あとはプログラムしていきましょう。
Swift5
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
self.setTabBarItem(index: 0, titile: "ホーム", image: UIImage(named: "tab_home_off")!, selectedImage: UIImage(named: "tab_home_on")!, offColor: UIColor.blue, onColor: UIColor.red)
self.setTabBarItem(index: 1, titile: "サーチ", image: UIImage(named: "tab_search_off")!, selectedImage: UIImage(named: "tab_search_on")!, offColor: UIColor.gray, onColor: UIColor.black)
UITabBarItem.appearance().setTitleTextAttributes([.font : UIFont.systemFont(ofSize: 11)], for: .normal)
UITabBarItem.appearance().setTitleTextAttributes([.font : UIFont.systemFont(ofSize: 11)], for: .selected)
}
func setTabBarItem(index: Int, titile: String, image: UIImage, selectedImage: UIImage, offColor: UIColor, onColor: UIColor) -> Void {
let tabBarItem = self.tabBarController?.tabBar.items![index]
tabBarItem!.title = titile
tabBarItem!.image = image.withRenderingMode(.alwaysOriginal)
tabBarItem!.selectedImage = selectedImage.withRenderingMode(.alwaysOriginal)
tabBarItem!.setTitleTextAttributes([ .foregroundColor : offColor], for: .normal)
tabBarItem!.setTitleTextAttributes([ .foregroundColor : onColor], for: .selected)
}
}
Objective-C
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
}
- (void)viewWillAppear:(BOOL)animated {
[super viewWillAppear:animated];
[self setTabBarItemWithIndex:0 title:@"ホーム" offImage:[UIImage imageNamed:@"tab_home_off"] onImage:[UIImage imageNamed:@"tab_home_on"] offColor:[UIColor blueColor] onColor:[UIColor redColor]];
[self setTabBarItemWithIndex:1 title:@"サーチ" offImage:[UIImage imageNamed:@"tab_search_off"] onImage:[UIImage imageNamed:@"tab_search_on"] offColor:[UIColor grayColor] onColor:[UIColor blackColor]];
[[UITabBarItem appearance] setTitleTextAttributes:@{ NSFontAttributeName : [UIFont fontWithName:@"HelveticaNeue-Bold" size:10.0f]} forState:UIControlStateNormal];
[[UITabBarItem appearance] setTitleTextAttributes:@{ NSFontAttributeName : [UIFont fontWithName:@"HelveticaNeue-Bold" size:10.0f]} forState:UIControlStateSelected];
}
- (void)setTabBarItemWithIndex:(int)index
title:(NSString *)title
offImage:(UIImage *)offImage
onImage:(UIImage *)onImage
offColor:(UIColor *)offColor
onColor:(UIColor *)onColor{
UITabBarItem *tabBarItem = self.tabBarController.tabBar.items[index];
tabBarItem.title = title;
tabBarItem.image = [offImage imageWithRenderingMode:UIImageRenderingModeAlwaysOriginal];
tabBarItem.selectedImage = [onImage imageWithRenderingMode:UIImageRenderingModeAlwaysOriginal];
[tabBarItem setTitleTextAttributes:@{ NSForegroundColorAttributeName : offColor} forState:UIControlStateNormal];
[tabBarItem setTitleTextAttributes:@{ NSForegroundColorAttributeName : onColor} forState:UIControlStateSelected];
}
@end
プログラム自体はそこまで難しくありません。
tabBarController.tabBar.itemsから1つづつUITabBarItemを取り出して画像、文字、文字色を個別設定するようにしているだけです。
appearanceだけでの変更に比べると少し面倒ではありますが、カスタマイズ性を考えるとこの実装が一番です。ぜひお試しください。